Clean Code
- Descrição
- Currículo
- FAQ
- Revisões
As a developer, you should be able to write code which works – of course!
A lot of developers write bad code nonetheless – even though the code works. Because “working code” is not the same as “clean code”!
This course teaches you how to write clean code – code that is easy to read and understand by humans, not just computers!
In this course, you’ll learn what exactly clean code is and, more importantly, how you can write clean code. Because if your code is written in a clean way, it’s easier to read and understand and therefore easier to maintain.
Because it’s NOT just the computer who needs to understand your code – your colleagues and your future self needs to be able to understand it as well!
In this course, we’ll dive into all the main “pain points” related to clean code (or bad code – depending on how you look at it) and you will not just learn what makes up bad code but of course also how to turn it into clean code.
Specifically, you will learn about:
-
Naming “things” (variables, properties, classes, functions, …) properly and in a clean way
-
Common pitfalls and mistakes you should avoid when naming things
-
Comments and that most of them are bad
-
Good comments you might consider adding to your code
-
Code formatting – both horizontal and vertical formatting
-
Functions and how to limit the number of function parameters
-
How to write clean functions by focusing on “one thing”
-
How levels of abstraction help you split functions and keep them small
-
How to write DRY functions and avoid unexpected side effects
-
Avoiding deeply nested control structures with guards and by extracting functionality into functions
-
Errors and error handling as a replacement for if-statements
-
Objects & data containers/ data structures and why that differentiation could matter
-
Cohesion and how to write good (small!) classes
-
The Law of Demeter and why it matters for clean code
-
What the SOLID principles are and why they matter when it comes to writing clean code
-
Much more!
This course is a compilation of common patterns, best practices, principles and rules related to writing clean code.
In this course, you’ll learn about a broad variety of concepts, rules, ideas, thoughts and principles and by the end of course, you’ll have a good idea of what to keep in mind when it comes to writing clean code.
This is not a design patterns or general patterns course though – we will entirely focus on patterns, rules and concepts that help with writing clean code specifically.
All these concepts and rules are backed up by examples, code snippets and demos. And to ensure that you get the most out of this course, and you don’t just learn a bunch of theory which you forget soon after, there also are plenty of challenges for you to apply what you learned!
This course uses Python, JavaScript and TypeScript for code examples but you don’t need to know these languages to follow along and get a lot out of the course. In addition, the course does not focus on a specific programming style or paradigm (like functional programming, object-oriented programming etc) but instead covers general concepts and techniques which will always apply.
What are the course prerequisites?
-
Basic programming knowledge (no matter which language) is required!
-
You don’t need to know any specific programming language or programming paradigm to follow along
-
NO prior experience with writing clean code is required
-
1Welcome to the Course!Vídeo Aula
-
2What is "Clean Code"?Vídeo Aula
-
3Clean Code - Key Pain Points & How To Write Clean CodeVídeo Aula
-
4How Is This Course Structured?Vídeo Aula
-
5Course PrerequisitesVídeo Aula
-
6Clean Code & Strongly Typed LanguagesVídeo Aula
-
7About The Course Code ExamplesVídeo Aula
-
8Join Our Learning Community!Texto
-
9Functional, OOP, Procedural: The Course Concepts Always Apply!Vídeo Aula
-
10Clean Code, Principles & Patterns & Clean ArchitectureVídeo Aula
-
11Clean Code vs Quick CodeVídeo Aula
-
12Module & Course ResourcesTexto
-
13Course SetupTexto
-
14Module IntroductionVídeo Aula
-
15Why Good Names MatterVídeo Aula
-
16Choosing Good NamesVídeo Aula
-
17Casing Conventions & Programming LanguagesVídeo Aula
-
18Naming Variables & Properties - TheoryVídeo Aula
-
19Naming Variables & Properties - ExamplesVídeo Aula
-
20Naming Functions & Methods - TheoryVídeo Aula
-
21Naming Functions & Methods - ExamplesVídeo Aula
-
22Naming Classes - TheoryVídeo Aula
-
23Naming Classes - ExamplesVídeo Aula
-
24Exceptions You Should Be Aware OfVídeo Aula
-
25Common Errors & PitfallsVídeo Aula
-
26Demo Time!Vídeo Aula
-
27Your Challenge - ProblemVídeo Aula
-
28Your Challenge - SolutionVídeo Aula
-
29Module ResourcesTexto
-
30Module IntroductionVídeo Aula
-
31Bad CommentsVídeo Aula
-
32Good CommentsVídeo Aula
-
33What is "Code Formatting" Really About?Vídeo Aula
-
34Vertical FormattingVídeo Aula
-
35Formatting: Language-specific ConsiderationsVídeo Aula
-
36Horizontal FormattingVídeo Aula
-
37Your Challenge - ProblemVídeo Aula
-
38Your Challenge - SolutionVídeo Aula
-
39Module ResourcesTexto
-
40Module IntroductionVídeo Aula
-
41Analyzing Key Function PartsVídeo Aula
-
42Keep The Number Of Parameters Low!Vídeo Aula
-
43Refactoring Function Parameters - Ideas & ConceptsVídeo Aula
-
44When One Parameter Is Just RightVídeo Aula
-
45Two Parameters & When To RefactorVídeo Aula
-
46Dealing With Too Many ValuesVídeo Aula
-
47Functions With A Dynamic Number Of ParametersVídeo Aula
-
48Beware Of "Output Parameters"Vídeo Aula
-
49Functions Should Be Small & Do One Thing!Vídeo Aula
-
50Why "Levels of Abstraction" MatterVídeo Aula
-
51When Should You Split?Vídeo Aula
-
52Demo & ChallengeVídeo Aula
-
53Stay DRY - Don't Repeat YourselfVídeo Aula
-
54Splitting Functions To Stay DRYVídeo Aula
-
55Don't Overdo It - Avoid Useless ExtractionsVídeo Aula
-
56Understanding & Avoiding (Unexpected) Side EffectsVídeo Aula
-
57Side Effects - A ChallengeVídeo Aula
-
58Why Unit Tests Matter & Help A Lot!Vídeo Aula
-
59Module ResourcesTexto
-
60Module IntroductionVídeo Aula
-
61Useful Concepts - An OverviewVídeo Aula
-
62Introducing "Guards"Vídeo Aula
-
63Guards In ActionVídeo Aula
-
64Extracting Control Structures & Preferring Positive PhrasingVídeo Aula
-
65Extracting Control Structures Into FunctionsVídeo Aula
-
66Writing Clean Functions With Control StructuresVídeo Aula
-
67Inverting Conditional LogicVídeo Aula
-
68Embrace Errors & Error HandlingVídeo Aula
-
69Creating More Error GuardsVídeo Aula
-
70Extracting Validation CodeVídeo Aula
-
71Error Handling Is One Thing!Vídeo Aula
-
72Using Factory Functions & PolymorphismVídeo Aula
-
73Working with Default ParametersVídeo Aula
-
74Avoid "Magic Numbers & Strings"Texto
-
75Module SummaryVídeo Aula
-
76Module ResourcesTexto
-
77Module IntroductionVídeo Aula
-
78Important: This is NOT an OOP or "Patterns & Principles" Course!Vídeo Aula
-
79Objects vs Data Containers / Data StructuresVídeo Aula
-
80Why The Differentiation MattersVídeo Aula
-
81Classes & PolymorphismVídeo Aula
-
82Classes Should Be Small!Vídeo Aula
-
83Understanding "Cohesion"Vídeo Aula
-
84The "Law Of Demeter" And Why You Should "Tell, Not Ask"Vídeo Aula
-
85The SOLID PrinciplesVídeo Aula
-
86The Single-Responsibility-Principle (SRP) & Why It MattersVídeo Aula
-
87The Open-Closed Principle (OCP) & Why It MattersVídeo Aula
-
88The Liskov Substitution PrincipleVídeo Aula
-
89The Interface Segregation PrincipleVídeo Aula
-
90The Dependency Inversion PrincipleVídeo Aula
-
91Module ResourcesTexto
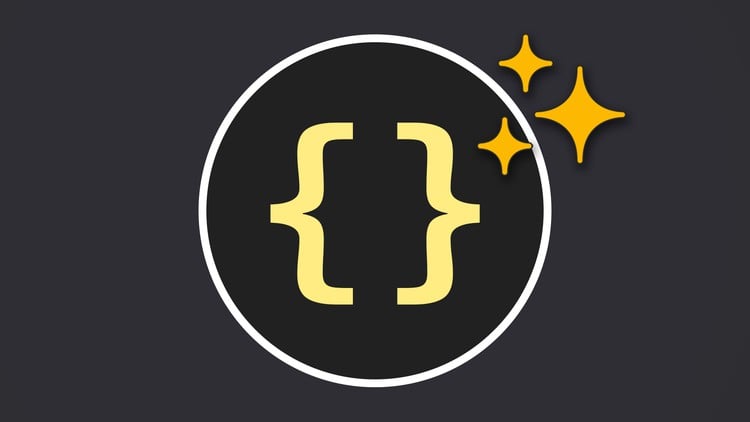