JavaScript: Understanding the Weird Parts (2024 Edition)
- Descrição
- Currículo
- FAQ
- Revisões
Javascript is the language that modern developers need to know, and know well. Truly knowing Javascript will get you a job, and enable you to build quality web and server applications.
NOTE: This course includes information on ECMAScript 6 (ES6) the next version of Javascript!
In this course you will gain a deep understanding of Javascript, learn how Javascript works under the hood, and how that knowledge helps you avoid common pitfalls and drastically improve your ability to debug problems. You will find clarity in the parts that others, even experienced coders, may find weird, odd, and at times incomprehensible. You'll learn the beauty and deceptive power of this language that is at the forefront of modern software development today.
This course will cover such advanced concepts as objects and object literals, function expressions, prototypical inheritance, functional programming, scope chains, function constructors (plus new ES6 features), immediately invoked function expressions (IIFEs), call, apply, bind, and more.
We'll take a deep dive into the source code of popular frameworks such as jQuery and Underscore to see how you can use your understanding of Javascript to learn (and borrow) from other's good code.
Finally, you'll learn the foundations of how to build your own Javascript framework or library.
What you'll learn in this course will make you a better Javascript developer, and improve your abilities in AngularJS, NodeJS, jQuery, React, Ember, MongoDB, and all other Javascript-based technologies!
Learn to love Javascript, and code in it well.
Note: In this course you'll also get downloadable source code. You will often be provided with 'starter' code, giving you the base for you to start writing your code, and 'finished' code to compare your code to.
-
7Conceptual Aside: Syntax Parsers, Execution Contexts, and Lexical EnvironmentsVídeo Aula
-
8Conceptual Aside: Name/Value Pairs and ObjectsVídeo Aula
-
9Downloading Source Code for This CourseTexto
-
10The Global Environment and The Global ObjectVídeo Aula
-
11The Execution Context - Creation and HoistingVídeo Aula
-
12Conceptual Aside: Javascript and 'undefined'Vídeo Aula
-
13The Execution Context - Code ExecutionVídeo Aula
-
14Conceptual Aside: Single Threaded, Synchronous ExecutionVídeo Aula
-
15Function Invocation and the Execution StackVídeo Aula
-
16Functions, Context, and Variable EnvironmentsVídeo Aula
-
17The Scope ChainVídeo Aula
-
18Scope, ES6, and letVídeo Aula
-
19What About Asynchronous Callbacks?Vídeo Aula
-
20Conceptual Aside: Types and JavascriptVídeo Aula
-
21Primitive TypesVídeo Aula
-
22Conceptual Aside: OperatorsVídeo Aula
-
23Operator Precedence and AssociativityVídeo Aula
-
24Operator Precedence and Associativity TableTexto
-
25Conceptual Aside: CoercionVídeo Aula
-
26Comparison OperatorsVídeo Aula
-
27Equality Comparisons TableTexto
-
28Existence and BooleansVídeo Aula
-
29Default ValuesVídeo Aula
-
30Framework Aside: Default ValuesVídeo Aula
-
31Objects and the DotVídeo Aula
-
32Objects and Object LiteralsVídeo Aula
-
33Framework Aside: Faking NamespacesVídeo Aula
-
34JSON and Object LiteralsVídeo Aula
-
35Functions are ObjectsVídeo Aula
-
36Function Statements and Function ExpressionsVídeo Aula
-
37Conceptual Aside: By Value vs By ReferenceVídeo Aula
-
38Objects, Functions, and 'this'Vídeo Aula
-
39Conceptual Aside: Arrays - Collections of AnythingVídeo Aula
-
40'arguments' and spreadVídeo Aula
-
41Framework Aside: Function OverloadingVídeo Aula
-
42Conceptual Aside: Syntax ParsersVídeo Aula
-
43Dangerous Aside: Automatic Semicolon InsertionVídeo Aula
-
44Framework Aside: WhitespaceVídeo Aula
-
45Immediately Invoked Functions Expressions (IIFEs)Vídeo Aula
-
46Framework Aside: IIFEs and Safe CodeVídeo Aula
-
47Understanding ClosuresVídeo Aula
-
48Understanding Closures - Part 2Vídeo Aula
-
49Framework Aside: Function FactoriesVídeo Aula
-
50Closures and CallbacksVídeo Aula
-
51call(), apply(), and bind()Vídeo Aula
-
52Functional ProgrammingVídeo Aula
-
53Functional Programming - Part 2Vídeo Aula
-
58Function Constructors, 'new', and the History of JavascriptVídeo Aula
-
59Function Constructors and '.prototype'Vídeo Aula
-
60Dangerous Aside: 'new' and functionsVídeo Aula
-
61Conceptual Aside: Built-In Function ConstructorsVídeo Aula
-
62Dangerous Aside: Built-In Function ConstructorsVídeo Aula
-
63Dangerous Aside: Arrays and for..inVídeo Aula
-
64Object.create and Pure Prototypal InheritanceVídeo Aula
-
65ES6 and ClassesVídeo Aula
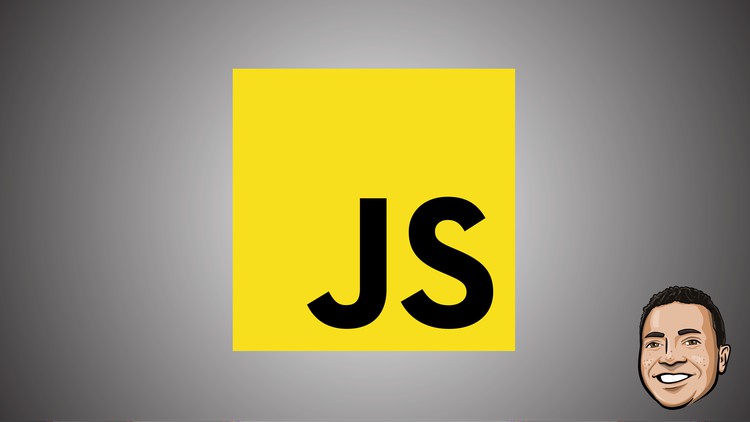