Test Java applications with JUnit 5, Mockito, Testcontainers
- Descrição
- Currículo
- FAQ
- Revisões
Unit Testing is a must-have skill and this video course is about unit testing.
If you take this video course, you will learn how to test your Java code using JUnit 5 and Mockito framework.
This video course is for beginners and you do not need to have any prior Unit testing knowledge to enrol in this course.
JUnit 5 in Different Java projects
There are different Java projects, build tools and development environments. In this course, you will learn how to create a new project and configure JUnit 5 support for different types of projects, using different development environments and build tools.
You will learn how to create a Unit test in:
-
IntelliJ IDEA and
-
Eclipse Development environments.
You will learn how to create a Unit test in:
-
Regular Java project,
-
Maven-based Java project,
-
Cradle-based Java project.
Course Overview
This video course teaches Unit and Integration testing with Java from the very beginning and covers many advanced topics as well. By the end of this course, you will learn:
-
JUnit 5 basics, and
-
JUnit 5 advanced topics.
Once you become familiar with JUnit 5, you will learn to use:
-
Test-Driven Development(TDD)
You will then learn to use another very popular Test framework for Java called:
-
Mockito
You will also learn how to write:
-
Spring Boot integration tests, and
-
Use Testcontainers to integrate real, containerized services(like databases) into your Java application tests, making sure your test scenarios are realistic without complex setup.
By the end of this course you will learn and be able to use all of the following:
-
Create Unit Tests in IntelliJ,
-
Create Unit Tests in Eclipse,
-
Run unit tests using Gradle,
-
Run Unit Tests using Maven,
-
Use @DisplayName annotation,
-
Use JUnit assertions,
-
Test for Exceptions,
-
Use Lifecycle methods (@BeforeAll, @BeforeEach, @AfterEach, @AfterAll),
-
Run unit tests in any order you need: (Random, Order by Name, Order by Index),
-
Disable Unit test,
-
Repeated Tests with @RepeatedTest annotation,
-
Parameterized tests with @Parameterized annotation
-
@ValueSource,
-
@MethodSource,
-
@CsvSource,
-
@CsvFileSource
-
-
Change Test Instance lifecycle with @TestInstance (PER_CLASS, PER_METHOD)
-
Learn to Mock objects with Mockito’s @Mock annotation,
-
Learn to user Mockito’s argument matches,
-
Mockito method stubbing,
-
Mockito Exception stubbing,
-
Verify method call,
-
Call Real Method,
-
Do nothing when a method is called,
-
Write integration tests for Spring Boot applications,
-
and more…
-
1IntroductionVídeo Aula
-
2Welcome from the instructorVídeo Aula
-
3What is a Unit Test?Vídeo Aula
-
4Why write Unit Test?Vídeo Aula
-
5The F.I.R.S.T PrincipleVídeo Aula
-
6Testing Code in IsolationVídeo Aula
-
7Testing PyramidVídeo Aula
-
8Quiz. Basics of Unit TestingQuestionário
-
9What is JUnit 5?Vídeo Aula
-
10JUnit and Build ToolsVídeo Aula
-
21IntroductionTexto
-
22Creating First Unit Test methodVídeo Aula
-
23Assertions and Assertion messageVídeo Aula
-
24Assertion MessageQuestionário
-
25Other assertionsVídeo Aula
-
26JUnit Test ExerciseTexto
-
27Exercise solution overviewVídeo Aula
-
28Lazy Assert MessagesVídeo Aula
-
29Naming Unit TestsVídeo Aula
-
30@DisplayName annotationVídeo Aula
-
31Test Method Code Structure. Arrange, Act, Assert.Vídeo Aula
-
32JUnit Test LifecycleVídeo Aula
-
33Lifecycle methods demoVídeo Aula
-
34Quiz - JUnit Test LifecycleQuestionário
-
35Disable Unit TestVídeo Aula
-
36Assert an ExceptionVídeo Aula
-
37@ParameterizedTest. Multiple Parameters with @MethodSource.Vídeo Aula
-
38@ParameterizedTest. Multiple parameters with @CsvSource.Vídeo Aula
-
39@ParameterizedTest + CSV fileVídeo Aula
-
40@ParameterizedTest + @ValueSource annotation.Vídeo Aula
-
41Repeated TestsVídeo Aula
-
42Methods Order - Random orderVídeo Aula
-
43Methods Order - Order by nameVídeo Aula
-
44Methods Order - Random by order indexVídeo Aula
-
45Order of Unit Test ClassesVídeo Aula
-
46Methods Execution Order - QuizQuestionário
-
47Test Instance Lifecycle - IntroductionVídeo Aula
-
48Changing Test Instance Lifecycle - example 1Vídeo Aula
-
49Test Instance Lifecycle Demo project overviewVídeo Aula
-
50Test Instance Lifecycle Demo Project ImplementationVídeo Aula
-
51Test Instance Lifecycle - QuizQuestionário
-
52Introduction to the Test Driven Development(TDD) in JavaVídeo Aula
-
53New project, Class, MethodVídeo Aula
-
54Creating UserServiceVídeo Aula
-
55Test Create User methodVídeo Aula
-
56Test User object contains first nameVídeo Aula
-
57Test Driven Development(TDD) - Refactor Test methodVídeo Aula
-
58Test Driven Development(TDD) - ExerciseVídeo Aula
-
59Test Driven Development(TDD) - Solution overviewVídeo Aula
-
60Check if user id is setVídeo Aula
-
61Assert throws ExceptionVídeo Aula
-
62TDD - Exercise 2Vídeo Aula
-
63Test Driven Development in Java - Quiz.Questionário
-
64Introduction to MockitoVídeo Aula
-
65Adding Mockto to a Java projectVídeo Aula
-
66Method under test overviewVídeo Aula
-
67Implementing UsersRepositoryVídeo Aula
-
68Injecting UsersRepository as DependencyVídeo Aula
-
69Mockito - Creating a Mock objectVídeo Aula
-
70Stubbing using built-in any() argument matcherVídeo Aula
-
71Mockito - Verify method callVídeo Aula
-
72Mockito - Exception stubbingVídeo Aula
-
73Creating EmailNotificationService classVídeo Aula
-
74Mockito - Stub a void method with ExceptionVídeo Aula
-
75Mockito - Do nothing when method is calledVídeo Aula
-
76Mockito - Call a real methodVídeo Aula
-
77Mockito - QuizQuestionário
-
78Code Coverage - IntroductionVídeo Aula
-
79Generating JUnit Code Coverage report with IntelliJVídeo Aula
-
80Export JUnit Code Coverage Report using IntelliJVídeo Aula
-
81Export JUnit Test Report using MavenVídeo Aula
-
82Jacoco - Maven Plugin for Code CoverageVídeo Aula
-
83Jacoco - Export Code Coverage Report in HTML formatVídeo Aula
-
84Code Coverage - QuizQuestionário
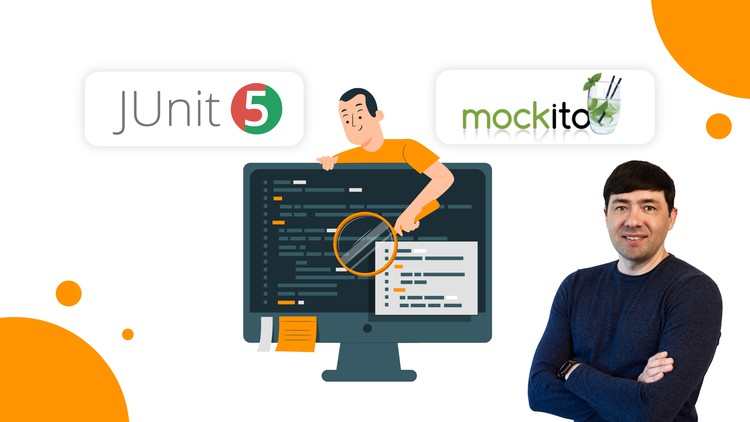