Unreal Engine 4 C++ The Ultimate Shooter Course
- Descrição
- Currículo
- FAQ
- Revisões
Please note that this course in done in Unreal Engine 4 and not in Unreal Engine 5.
This is the most comprehensive Unreal Engine tutorial series on the internet. You will create a shooter game complete with AAA quality gameplay mechanics. Learn the theory behind the gameplay mechanics of shooter games, then implement them in this beautiful game project. Topics covered are:
-
Character creation and movement
-
Input for PC and console controllers
-
Extensive use of Animation Blueprints (and Anim Instances, their C++ parents)
-
1D and 2D Blendspaces
-
Strafing
-
Turn-in-place
-
Aim Offsets
-
Inverse Kinematics
-
Animation Curves
-
Character lean when running
-
Crouching (with dynamic capsule resizing)
-
Turn hips while running
-
Weapon fire with recoil animations
-
Reloading
-
Weapon blast and impact particles
-
Weapon beam particles (smoke trails)
-
Bullet shell eject particles
-
Sound effects
-
Blending animations per bone, by bool, and by enum – play one animation with one part of the body (running or crouching) while simultaneously playing another animation with another part of the body (reload, aim, or weapon fire)
-
Attach and equip different weapons (pistols, submachine guns, assault rifles)
-
Automatic and semi-automatic gunfire
-
Move different gun parts (the clip/magazine, and pistol slide) during animations
-
Camera zoom while aiming
-
Dynamic crosshairs that spread in reaction to:
-
Character speed
-
Weapon fire
-
Aiming
-
Jumping
-
-
Different crosshairs per weapon
-
Widget components, showing:
-
Item names
-
Item types
-
Ammo counts
-
Item rarity
-
-
HUD animations
-
Full item inventory system
-
Data tables, in Blueprints and C++
-
Curves to control:
-
Item movement during pickup
-
Color and brightness pulse for material effects
-
-
Material creation, including:
-
Post-process materials
-
Dynamic material instances
-
Setting material properties from C++
-
Driving material properties with curves
-
Material functions
-
Blending materials together
-
Outline effects
-
Glow/pulse effects
-
-
Retargeting animations
-
Retargeting whole Animation Blueprints
-
Numerous gameplay algorithms
-
Use of data structures, including:
-
structs
-
enums
-
arrays
-
maps
-
and more
-
-
Animation montages
-
Anim Notifies for sounds, weapon trails, and custom notifies
-
Sync markers for footsteps and sync groups
-
The course comes with a huge amount of assets, including:
-
Sounds
-
Textures
-
Particle effects
-
Meshes
-
-
Delegates
-
Interfaces
-
Dynamic footsteps that spawn different sounds and particle systems depending on the surface type
-
Physical materials and surface types
-
Niagra particle systems
-
Line traces
-
Enemy AI
-
Behavior Trees and Blackboard Components
-
Multiple enemy types with varying levels of Health, Damage, Size and Movement Speed
-
Headshot damage, with:
-
Higher damage for headshots
-
Number widgets that pop up and animate with bullet hits
-
Different colored numbers for headshots
-
-
Character and enemy health bars
-
Enemy patrol, agro, chase and attack player
-
Death mechanics
-
Stun mechanics
-
Melee attacks with melee weapon trails
-
Explosives that cause damage and death
-
Level prototyping
-
Creating full levels based on our prototypes using professional assets
-
Light baking
-
Post-process effects
-
1IntroductionVídeo Aula
Everything you learn in this course will translate to Unreal Engine 5. Unreal Engine 5 uses the same code base as Unreal Engine 4, so when UE5 is released, you will be prepared to use the world's leading game development engine!
Take the most comprehensive game development course on the internet! Learn techniques to get all of the shooter gameplay mechanics into your game that AAA titles have.
-
2Install an IDEVídeo Aula
All C++ programmers need an IDE (integrated development environment). We go over three options, depending on your system. Each of these are free to download and use.
-
3Install Unreal EngineVídeo Aula
Unreal Engine is free to download! Create an Epic Games account and get the world's leading game engine so we can start building this game!
-
4C++ RefresherVídeo Aula
These are important concepts you must understand to use Unreal Engine's C++ code base. Don't worry, everything in this course will be explained as we code it. Ask other students on the discord server if you're unsure about something!
-
5Quiz on C++ ConceptsQuestionário
This quiz will ask you a few questions to make sure you understand some important C++ concepts.
-
6Reflection and Garbage CollectionVídeo Aula
Unreal Engine has a reflection system that makes it easier for us to program dynamically. This lecture covers the basics of garbage collection and reflection.
-
7How to Get HelpVídeo Aula
We learn how to get help from various sources, how to get the assets for this course, and how to download the finished project from the GitHub page.
-
8Quiz on Getting HelpQuestionário
This quiz is designed to make sure you understand the best ways to get help during this course.
-
9Project SetupVídeo Aula
We create our project and set it up with a blank new level.
-
10Character ClassVídeo Aula
We create our character class based on ACharacter. We're starting this shooter character from scratch!
-
11UE_LOG Format String - IntVídeo Aula
UE_LOG is an important tool for Unreal Engine C++ developers. We brush up on how to send messages to the Output Log.
-
12UE_LOG Format SpecifiersVídeo Aula
Format specifiers allow us to customize our log message and include values from data in our game.
-
13UE_LOG with FStringVídeo Aula
We must be able to manipulate strings using Unreal Engine's FString type. We gets some practice with that in this video.
-
14Camera Spring ArmVídeo Aula
We add a Spring Arm Component, which we will use to attach a camera.
-
15Follow CameraVídeo Aula
We add a camera that is attached to the Spring Arm, which follows the Character.
-
16Controllers and InputVídeo Aula
We go into detail about what the Controller is and its relationship to the other classes in Unreal Engine.
-
17Quiz on the Controller ClassQuestionário
This quiz checks to make sure you understand some basic concepts related to the controller.
-
18Move Forward and RightVídeo Aula
We learn how to move the Character back and forth, as well as side to side with user input.
-
19Delta TimeVídeo Aula
We discuss the theory behind DeltaTime and why it's important to use this quantity each frame in order to keep our gameplay consistent and smooth.
-
20Turn at RateVídeo Aula
We create rate-based movement to handle turning with the use of key and button presses.
-
21Mouse Turning and JumpingVídeo Aula
We implement the ability to turn using the mouse, in addition to key presses. We also implement the ability to jump.
-
22Adding a MeshVídeo Aula
We add an actual Mesh to our Character, so we can have a visual representation in the world! It's getting serious!
-
23Quiz on Section 2Questionário
Quiz on Section 2
-
24The Anim InstanceVídeo Aula
We create the Anim Instance, the class from which the Animation Blueprint is derived. This is where we will access some data from the C++ side to expose to the Animation Blueprint.
-
25The Animation BlueprintVídeo Aula
We create the Animation Blueprint based on the Anim Instance class, and assign it to the Character.
-
26Run AnimationsVídeo Aula
We add run animations to our Ground Locomotion state machine in the Animation Blueprint.
-
27Trimming AnimationsVídeo Aula
-
28Rotate Character to MovementVídeo Aula
We access the Character Movement Component and configure some of its properties in order to customize our Character's behavior.
-
29Quiz on Character MovementQuestionário
Quiz to test your comprehension of Character Movement.
-
30Fire Weapon FunctionVídeo Aula
We create a function called FireWeapon and bind it to the left mouse button and the right console controller button. For now, it simply prints a message to the Output Log.
-
31Shooting Sound EffectsVídeo Aula
We create a Sound Cue using assets from the GitHub page, randomizing 10 different gun shots. We then play the Sound Cue in the FireWeapon function.
-
32Shooting ParticlesVídeo Aula
We add a socket to the Character's skeleton and position it at the gun barrel. We then spawn a weapon fire particle system at the barrel socket when firing the weapon.
-
33Shooting AnimationVídeo Aula
We create an Animation Montage with a weapon fire animation, assign it a slot, then play this montage when firing the weapon.
-
34Blending Shooting AnimationsVídeo Aula
We use a Layered Blend Per Bone node to play the shooting animation on the upper body while Ground Locomotion is played on the lower body.
-
35Line Tracing for Bullet HitsVídeo Aula
We use a line trace to detect bullet hits and get a bullet hit location.
-
36Quiz on Line TracesQuestionário
Quiz on Line Traces
-
37Impact ParticlesVídeo Aula
We use the line trace hit location to spawn bullet impact particles.
-
38Beam ParticlesVídeo Aula
We use beam particles to create a smoke trail for firing the weapon.
-
39Socket OffsetVídeo Aula
We add a socket offset to control the camera location relative to the character location.
-
40HUD Class and CrosshairsVídeo Aula
We create a HUD class and add crosshairs to the screen.
-
41Directing Rifle ShotsVídeo Aula
We add the ability to shoot our bullet in the direction we're aiming.
-
42Quiz on Directing Rifle ShotsQuestionário
Quiz on the Directing Rifle Shots lecture.
-
43Trace from Gun BarrelVídeo Aula
We perform a second trace, from the gun barrel to the trace end location, in order to check for objects between the barrel and the hit location.
-
44Refactor Beam End CodeVídeo Aula
We refactor the code in FireWeapon so the Beam End location is calculated in its own neat little function.
-
45Movement Offset YawVídeo Aula
We calculate the difference between the yaw rotation of our movement direction and the yaw rotation of our aim direction, in order to get a quantity to use for strafing animations.
-
46Strafing BlendspaceVídeo Aula
We create a strafing Blendspace to use our MovementOffsetYaw variable and strafe depending on your run direction.
-
47Quiz on StrafingQuestionário
A short quiz to test your comprehension of strafing mechanics.
-
48Jog Start BlendspaceVídeo Aula
We need a different jog start animation depending on the direction we move. We implement that in this video.
-
49Jog Stop BlendspaceVídeo Aula
You were challenged to create a jog stop blendspace. We go over the solution to this challenge.
-
50Smoothing Character MovementVídeo Aula
We tweak some parameters so the Character moves smoothly and skids to a halt, working well with the jog stop animations.
-
51Quiz on Section 3Questionário
We now have an animated shooter Character! This quiz will test a few knowledge points you've learned from this section.
-
52Zooming Field of ViewVídeo Aula
We change the field of view while aiming the weapon.
-
53Aiming Zoom InterpolationVídeo Aula
We use interpolation to smoothly change the field of view.
-
54Quiz on Zoom InterpolationQuestionário
A quiz to test your comprehension of the Zoom Interpolation mechanic.
-
55Aiming PoseVídeo Aula
We blend between poses to get the Character to lift the weapon while aiming.
-
56Aiming State MachineVídeo Aula
We create an Aiming State Machine so the Character can transition between hip and aiming poses.
-
57Aim Look SensitivityVídeo Aula
We lower the look sensitivity while aiming to make it easier to zero in on a target.
-
58Crosshair Spread VelocityVídeo Aula
We create a variable to affect the spreading of the crosshairs.
-
59Spreading the CrosshairsVídeo Aula
We implement the spreading of the crosshairs.
-
60Crosshair In Air FactorVídeo Aula
We add a factor for affecting the spreading of crosshairs while in the air.
-
61Crosshair Aim FactorVídeo Aula
We make the crosshairs shrink while aiming the weapon.
-
62Bullet Fire Aim FactorVídeo Aula
We spread the crosshairs slightly with the firing of the weapon.
-
63Quiz on Dynamic CrosshairsQuestionário
A short quiz to test your comprehension of the Dynamic Crosshairs mechanic.
-
64Warning: Factory Level is LARGETexto
-
65New Level AssetsVídeo Aula
We look for some free assets on the Unreal Engine marketplace. This section is purely for the visuals of our level. Please make sure you have enough disk space for any assets you download!
-
66Automatic FireVídeo Aula
We use timers to implement automatic gun fire.
-
67Quiz on Automatic Fire.Questionário
A short quiz on Automatic Fire.
-
68Jumping AnimationsVídeo Aula
We implement jumping, making use of Apply Additive to use an additive animation while landing.
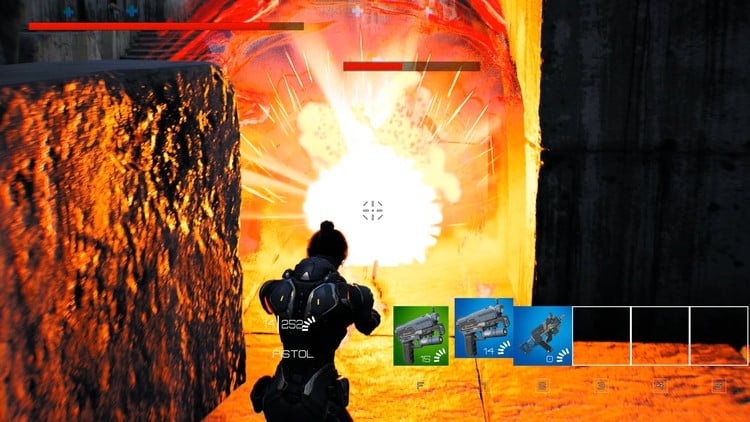